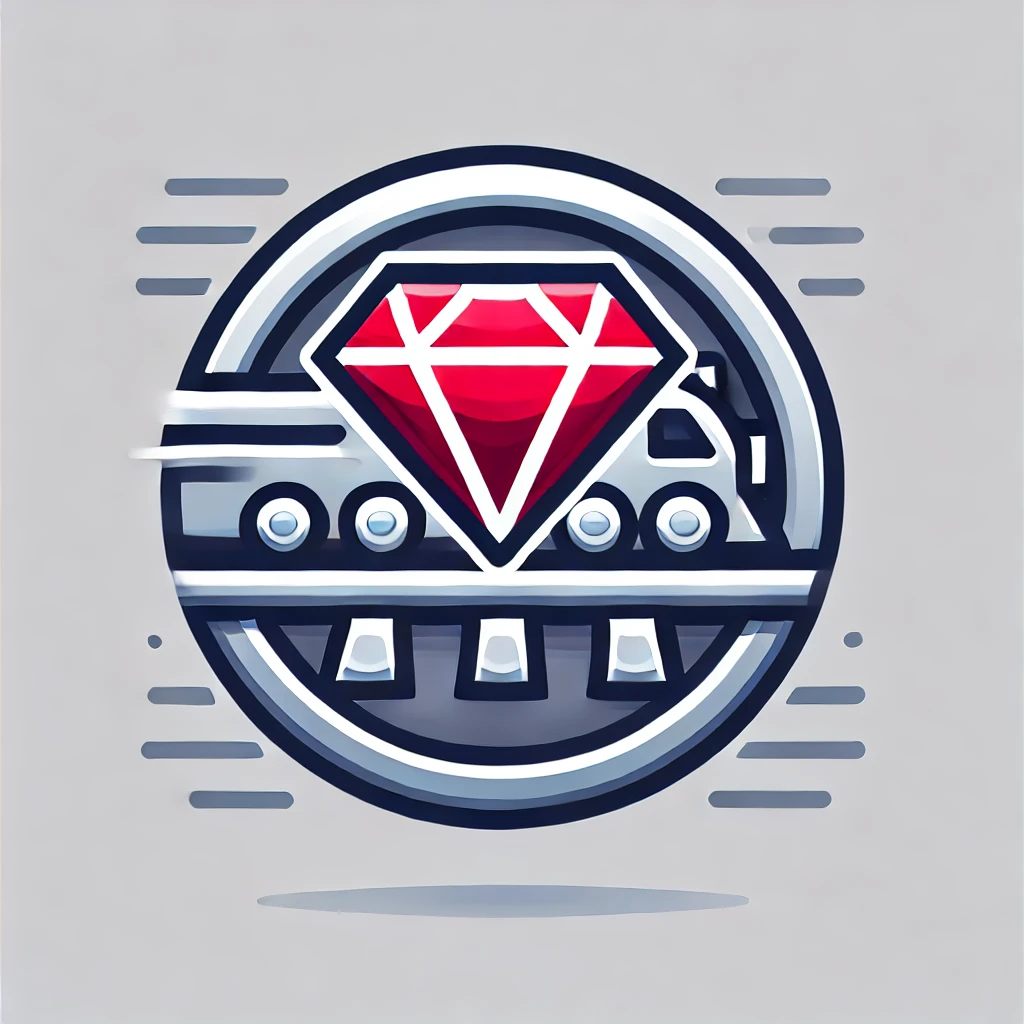
Ruby on Rails Development
Building MVPs, adding feature to existing apps, and scaling your SaaS. We do it all.
Recent posts from the blog:
- Technical Debt is a Project Killer
- How I Blog
- Get comfy in the Ubuntu terminal
- Dragon Astronauts Store Launch! 🚀🐉
- Why you should use Docker in development.
- Why we unit test.
- What is a Ruby block?
- Notes on the Rails 8 Keynote
- Ruby blocks, procs, lambdas and closures
- Better, faster code with test driven development
- ChatGPT is still making lots of mistakes.
- The Power of Play for better Team Meetings
- Software Estimates and Safety Margins
- What to do when you're laid off.
- Should I fix the terrible app I inherited?